Subgraphs: How to query Uniswap V2 subgraph
TLDR:
- Demonstrates how to query real-time and historical liquidity, swaps, volumes, and token price data in Uniswap V2 using a Chainstack elastic subgraph.
- Offers practical GraphQL queries for common DeFi use cases – e.g., filtering by token pair, setting USD thresholds, fetching daily/hourly volumes, and monitoring top liquidity providers.
- Provides a Python-based approach to automate subgraph queries, store results, and integrate them into data pipelines or trading dashboards.
Main article
In this tutorial, we will explore how to query Uniswap V2, an already-deployed subgraph on Chainstack. Subgraphs are a powerful solution for indexing blockchain data. You can access major DeFi protocols such as Uniswap, Curve, and PancakeSwap through elastic subgraphs. No heavy deployment or programming needed.
Query DeFi subgraphs on Chainstack
Start for free and get your app to production levels immediately. No credit card required. You can sign up with your GitHub, X, Google, or Microsoft account.
Introduction
Chainstack subgraphs simplify the extraction and processing of data from archive nodes, allowing for easy filtering and querying using GraphQL. Without subgraphs, Web3 developers and users would need to aggregate, process, and filter blockchain data themselves, which involves parsing vast amounts of raw transaction data.
We will discuss a subgraph for Uniswap V2. For more information on setting up dedicated custom subgraphs, please refer to our detailed tutorial here.
Uniswap V2 subgraph
The Uniswap V2 subgraph is an already-deployed subgraph that monitors and indexes data from Uniswap V2 smart contracts. You can explore the subgraph schema using the Explorer tool on the GraphiQL page of the subgraph, accessible via the GraphQL UI URL. Additionally, the GraphiQL page offers a convenient tool for testing different queries directly in the browser.
Automated liquidity protocol
Uniswap V2 is an automated liquidity protocol powered by a constant product formula and implemented in a system of non-upgradeable smart contracts on the Ethereum blockchain.
Each Uniswap smart contract, or pair, manages a liquidity pool made up of reserves of two ERC-20 tokens.
Anyone can become a liquidity provider (LP) for a pool by depositing an equivalent value of each underlying token in return for pool tokens. These tokens track pro-rata LP shares of the total reserves, and can be redeemed for the underlying assets at any time.
According to the schema, we can interact with the following entities, among others:
- Liquidity positions;
- Pairs;
- Swaps;
- Tokens;
- Transactions.
Additionally, there are several historical data entities that might be useful:
- Liquidity positions snapshots;
- Hourly and daily pair data;
- Daily token data.
Each entity contains different sets of parameters, fields and nested entities.
Querying subgraph
There are multiple ways to query the subgraph. You can use GraphiQL, as mentioned earlier, or the command-line tool curl
, along with many other HTTP packages or GraphQL clients implemented in various programming languages. For the examples below, we will use the requests
package, a popular and user-friendly HTTP client library for Python.
We will write a Python function to query the subgraph. GraphQL queries are stored as separate files. You can find all the scripts and queries in the GitHub repository.
Lowercased addresses
Note that addresses used in the subgraph are lowercased. Using checksummed addresses or addresses with uppercase letters may result in empty or incorrect outputs.
Prerequisites
-
Log in to your Chainstack account and add an elastic Uniswap V2 subgraph in the Ethereum Mainnet.
-
Open your terminal (Command Prompt for Windows or Terminal for macOS/Linux) and run the following command to clone the GitHub repository to your current directory.
-
Set up your Python virtual environment.
macOS/Linux:
Windows:
-
With the virtual environment activated, run the following command to install the required dependencies.
-
Create the environment variable file (
.env
) and paste your Chainstack endpoint URL there.
Python main.py
Let’s first create a main function that will run all the queries in a loop. We’ll start by loading the configuration file (.env
).
In our project, we store queries as separate .graphql
files in the Queries
folder. We will read each query file, execute it, and store its result in the Outputs
folder.
Meta queries
Get subgraph details. We can check the latest block synced to the subgraph by querying _meta
. Additionally, there is the deployment
field, which provides us with the unique identifier for the subgraph deployment. Append it to https://ipfsgw.com/ipfs/
to check the subgraph manifest content. hasIndexingErrors
indicates where there have been any indexing errors.
Queries for traders
Swaps
Get latest swaps. Here, we query the swaps
entity with a few parameters: first
to limit the response size, orderBy
and orderDirection
to sort swaps by time in descending order.
Get latest swaps for pair of tokens. To obtain a pair address, you can call the getPair
function of the UniswapV2 Factory Contract (no gas is needed). You can also query the subgraph using specific conditions, such as traded pairs with USDC (this will be covered later in the tutorial). Use where
to filter the results.
Get the latest swaps with threshold. In this case, we need to use the amountUSD
field. In the Explorer, we can see there is a special field for this called amountUSD_gte
. This field compares values to see if they are greater than or equal to a specified input.
Token prices
Get token price. To do this, we need to query the token
entity with the argument id
(token address). Since prices are in ETH, we should also include ethPrice
from the bundles
entity.
Get historical token prices. Here, we can use tokenDayDatas
, which stores historical data sets for all tokens. Prices are in USD.
Trading volume
Get hourly trading volume of pair. There is both daily and hourly data available. For our example, we will check the hourly data for the USDC/WETH pair (id
) since July 1, 2024 (hourStartUnix_gte
).
Get daily trading volume of a pair. The same approach for querying daily data. Here, we retreive data for the last 7 days.
Queries for liquidity providers
Pairs
Get largest pairs. The largest pairs have the largest reserves converted to USD. Sort by reserveUSD
in descending order.
Get recently created pools. The most recent pools have the highest block number. Sort by createdAtBlockNumber
in descending order.
Liquidity positions and DEX metrics
Get largest liquidity positions in pair. We can filter by the id
of the pair to get data for a specific pair. To find the largest positions, sort by liquidityTokenBalance
in descending order.
Get historical data on liquidity positions in pair. Snapshots store historical data for liquidity positions. Sort by block
in descending order, filtering for a specific pair.
Get Uniswap performance. Uniswap data is accumulated and condensed into daily statistics in the uniswapDayDatas
entity.
Conclusion
In this tutorial, we explored various entities such as swaps, tokens, and liquidity positions, and how to query them using GraphQL and Python. Additionally, we provided examples of querying historical data and filtering results based on specific conditions. By following this tutorial, you can effectively interact with and analyze data from Uniswap V2 using Chainstack subgraphs.
About author
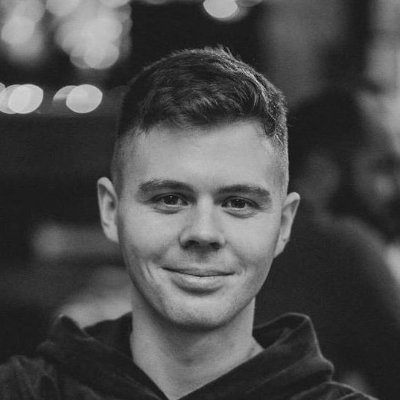
Anton Sauchyk
Developer Advocate @ Chainstack
Multiple years of software development and Web3 expertise. Creator of the open-source Compare Dashboard for RPC provider performance benchmarking. Core contributor to the DevEx team’s pump.fun trading bot. Author of technical tutorials on EVM blockchains, Solana, TON and Subgraphs.